How to pass API Gateway query parameters to AWS Lambda?
- Authors
- Name
- Tomasz Łakomy
- @tlakomy
Tired of using AWS Console? 🤕
Time to boost your productivity with Cloudash — an AWS desktop client.
First - make sure that you have set up Lambda proxy integration in API Gateway. Once that's done, you can access API Gateway parameters such as queryStringParameters
or pathParameters
in your Lambda function by using the event
object.
Here's a Node.js example:
Copy code
exports.handler = async (event) => {
// Get the querystring and route parameters from the event
const queryParams = event.queryStringParameters;
const routeParams = event.pathParameters;
// Use the querystring and route parameters in your logic
// ...
// Return a response
return {
statusCode: 200,
body: JSON.stringify({
// Data to return in the response
}),
};
};
The following example from AWS docs shows the structure of an event that API Gateway sends to a Lambda proxy integration:
{
"resource": "/my/path",
"path": "/my/path",
"httpMethod": "GET",
"headers": {
"header1": "value1",
"header2": "value2"
},
"multiValueHeaders": {
"header1": ["value1"],
"header2": ["value1", "value2"]
},
"queryStringParameters": {
"parameter1": "value1",
"parameter2": "value"
},
"multiValueQueryStringParameters": {
"parameter1": ["value1", "value2"],
"parameter2": ["value"]
},
"requestContext": {
"accountId": "123456789012",
"apiId": "id",
"authorizer": {
"claims": null,
"scopes": null
},
"domainName": "id.execute-api.us-east-1.amazonaws.com",
"domainPrefix": "id",
"extendedRequestId": "request-id",
"httpMethod": "GET",
"identity": {
"accessKey": null,
"accountId": null,
"caller": null,
"cognitoAuthenticationProvider": null,
"cognitoAuthenticationType": null,
"cognitoIdentityId": null,
"cognitoIdentityPoolId": null,
"principalOrgId": null,
"sourceIp": "IP",
"user": null,
"userAgent": "user-agent",
"userArn": null,
"clientCert": {
"clientCertPem": "CERT_CONTENT",
"subjectDN": "www.example.com",
"issuerDN": "Example issuer",
"serialNumber": "a1:a1:a1:a1:a1:a1:a1:a1:a1:a1:a1:a1:a1:a1:a1:a1",
"validity": {
"notBefore": "May 28 12:30:02 2019 GMT",
"notAfter": "Aug 5 09:36:04 2021 GMT"
}
}
},
"path": "/my/path",
"protocol": "HTTP/1.1",
"requestId": "id=",
"requestTime": "04/Mar/2020:19:15:17 +0000",
"requestTimeEpoch": 1583349317135,
"resourceId": null,
"resourcePath": "/my/path",
"stage": "$default"
},
"pathParameters": null,
"stageVariables": null,
"body": "Hello from Lambda!",
"isBase64Encoded": false
}
Tired of switching between AWS console tabs? 😒
Cloudash provides clear access to CloudWatch logs and metrics, to help you make quicker decisions.
Try it for free:
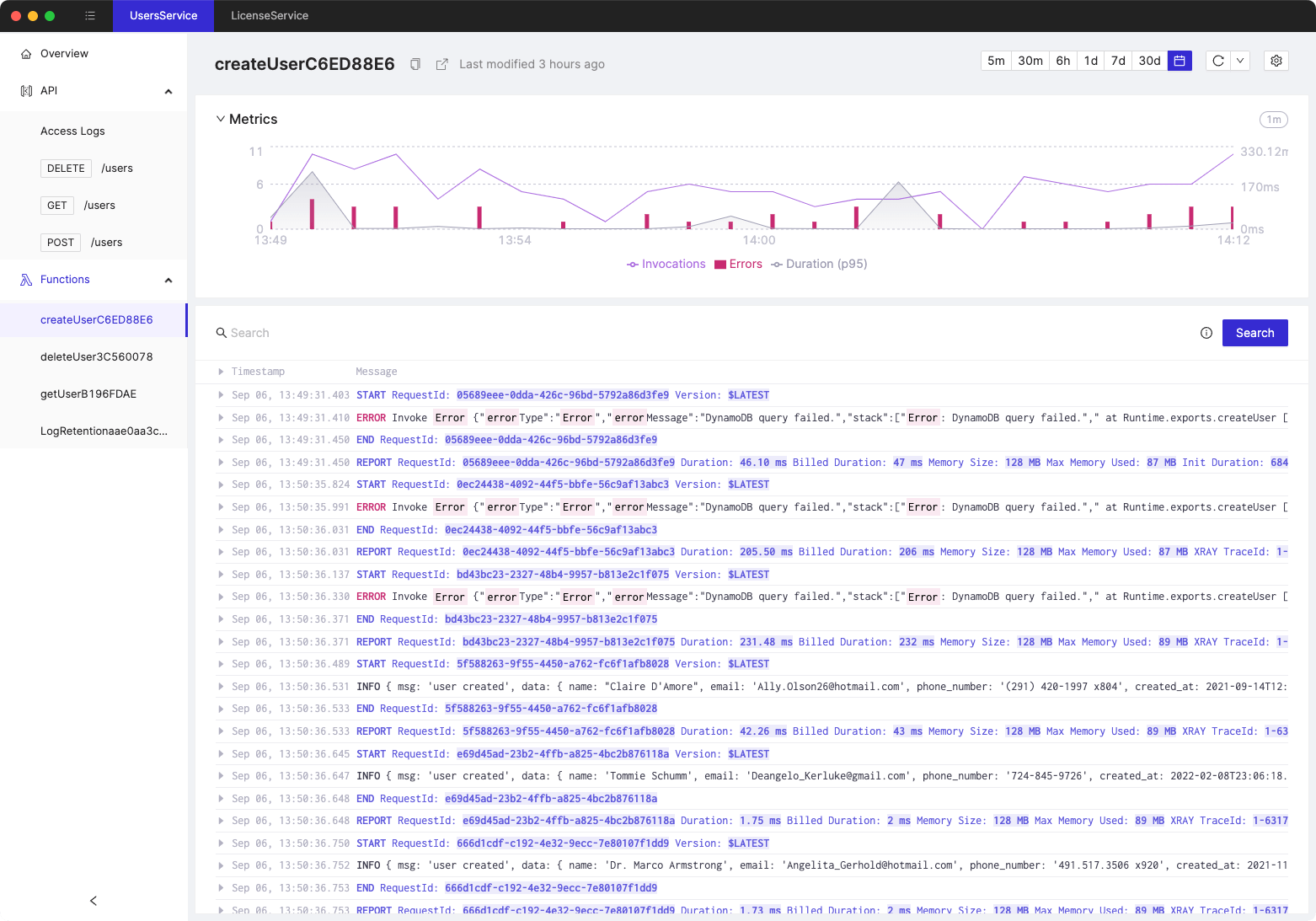